Smart contract security requires multiple protective measures. Developers should implement reentrancy guards, utilize SafeMath libraries for arithmetic operations, and follow the Checks-Effects-Interactions pattern. Role-based access controls limit function accessibility to authorized users only. External dependencies should come from reputable, audited sources. Regular security audits combining automated tools and manual reviews identify vulnerabilities before deployment. Building upgrade paths enables contracts to adapt to evolving threats. These foundational practices form just the beginning of a thorough security strategy.
Key Takeaways
- Implement reentrancy guards and update state variables before external calls to prevent attackers from draining funds.
- Use SafeMath libraries or Solidity 0.8.0+ to protect against integer overflow vulnerabilities in financial calculations.
- Follow the Checks-Effects-Interactions pattern by validating conditions first and making external calls last.
- Establish role-based access controls to restrict function access and minimize potential attack surfaces.
- Conduct comprehensive security audits combining automated tools like Slither with expert manual code review.
Understanding Reentrancy Vulnerabilities and Prevention Methods
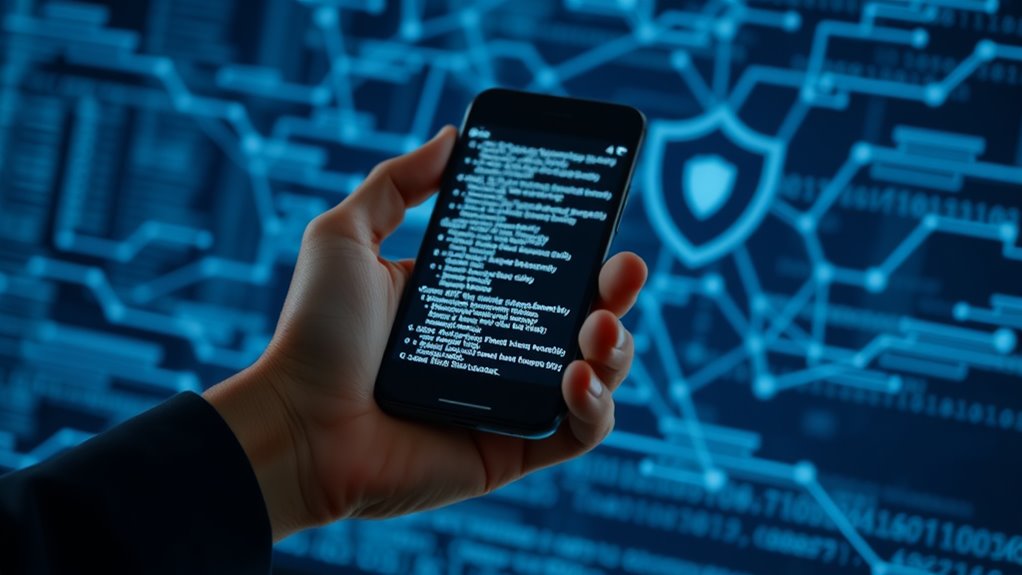
While smart contracts offer revolutionary potential for secure transactions, they remain susceptible to various attack vectors, with reentrancy vulnerabilities ranking among the most dangerous.
These attacks occur when malicious contracts repeatedly call functions in vulnerable contracts before initial executions complete, potentially draining all funds.
Contracts become vulnerable when they make external calls before updating their state. The infamous DAO Hack of 2016, which resulted in $50 million in losses, exemplifies the devastating impact of such vulnerabilities.
Unguarded external calls precede state updates: a $50 million lesson from the 2016 DAO catastrophe.
To prevent reentrancy attacks, developers should update state variables before making external calls and implement reentrancy guards.
Using modifiers like ‘noReentrant’ and maintaining sequential execution can provide additional protection. Regular security audits using tools like Slither or Mythril help identify potential vulnerabilities before deployment.
Simplicity in contract design greatly reduces vulnerability risk.
Implementing SafeMath Libraries to Prevent Integer Overflows
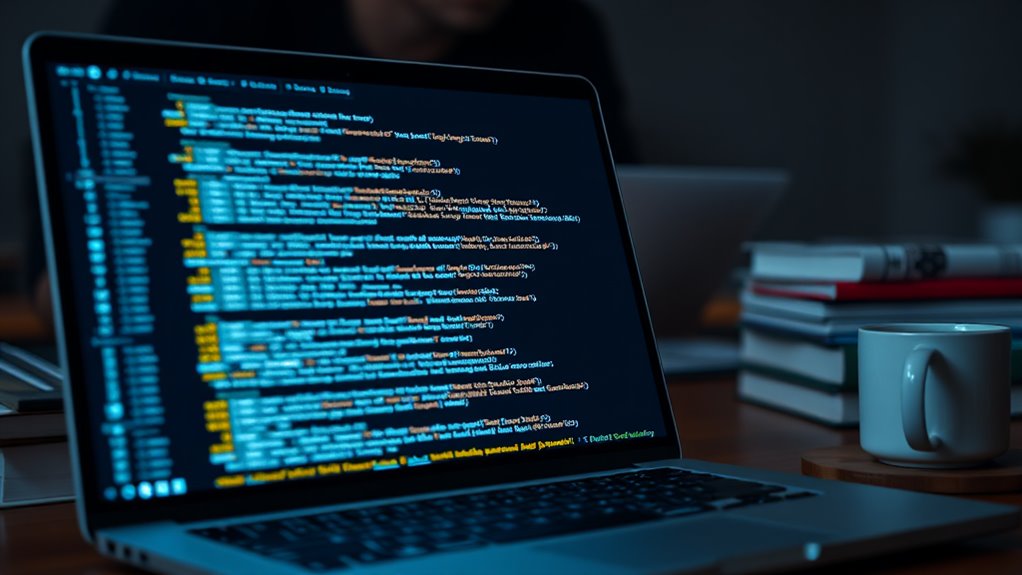
How often do developers overlook the risks of basic arithmetic operations in smart contracts? Integer overflows and underflows represent significant vulnerabilities that can lead to devastating financial losses when exploited.
SafeMath libraries provide essential protection by offering secure functions like `add()`, `sub()`, and `mul()` that automatically check for potential overflows. Implementing these libraries is straightforward: import the library, add a using directive (`using SafeMath for uint;`), and replace standard operations with SafeMath equivalents.
While Solidity 0.8.0 introduced built-in overflow checks, SafeMath remains vital for contracts built on earlier versions. Developers should either utilize SafeMath libraries when working with older Solidity versions or leverage the built-in protections of newer versions to guarantee arithmetic operations cannot be exploited.
Establishing Effective Role-Based Access Controls
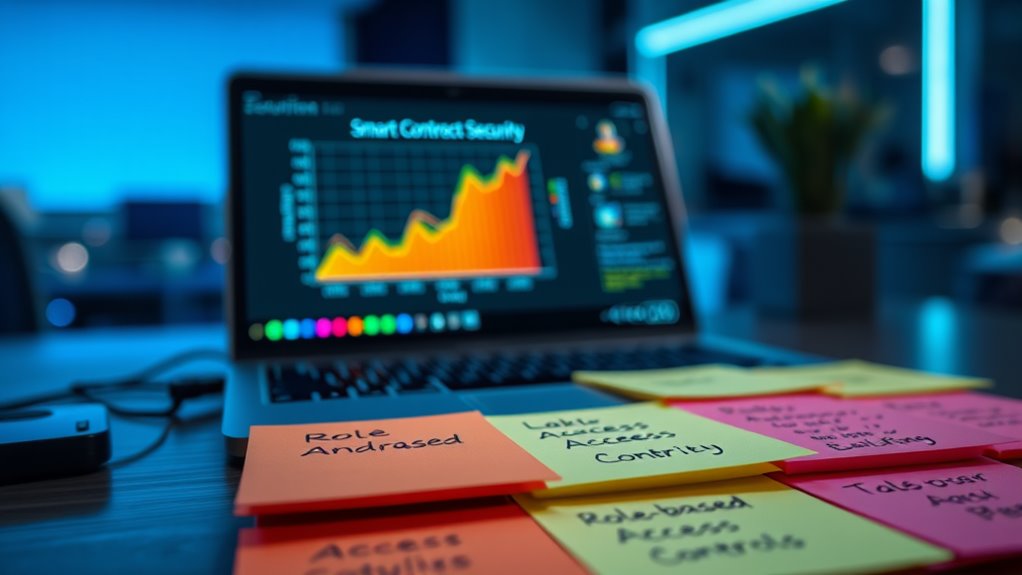
A well-designed smart contract requires a permission hierarchy implementation that clearly defines which roles can access specific functions and features.
Smart contract developers should establish distinct layers of access, from basic user permissions to privileged administrative capabilities, ensuring sensitive operations remain protected from unauthorized execution.
Dynamic rights management allows for roles to be assigned, modified, or revoked as needed, creating flexibility while maintaining security boundaries that adapt to organizational changes over time.
Permission Hierarchy Implementation
Establishing proper permission structures stands as a critical foundation for smart contract security. Implementing Role-Based Access Control (RBAC) assigns specific permissions to users based on their responsibilities, while adhering to the Principle of Least Privilege minimizes potential attack surfaces.
Developers should leverage established libraries like OpenZeppelin to avoid reinventing secure access control mechanisms.
- Use access modifiers (like `onlyOwner` or `onlyAdmin`) to restrict function access to authorized roles
- Implement multi-signature requirements for high-value transactions or critical protocol changes
- Document all permission structures clearly for transparency and future maintenance
- Test role-based systems thoroughly to guarantee they function as intended
- Consider timelocks for sensitive operations to provide time for community review
Additionally, maintaining strong passwords and employing multi-factor authentication can further bolster the security of smart contracts against unauthorized access.
Dynamic Rights Management
Effective dynamic rights management forms the cornerstone of secure smart contract development, enabling developers to control who can execute specific functions while adapting to changing organizational needs. By implementing role-based access controls (RBAC), smart contracts can restrict system access to authorized users based on their organizational roles.
Access Control Type | Implementation Method | Security Benefit |
---|---|---|
Single Owner | `onlyOwner` modifier | Simple management |
Role-Based | Function modifiers | Granular permissions |
Multi-Signature | Wallet integration | Distributed control |
Time-Locked | Delayed execution | Prevents rushed actions |
Attribute-Based | Conditional checks | Context-aware security |
While public blockchains present challenges for post-deployment permission changes, developers can utilize off-chain mechanisms or multi-step processes to achieve dynamic management. Regular audits and security reviews help identify vulnerabilities in access control implementation, ensuring permissions remain appropriate as organizational structures evolve. Additionally, understanding smart contracts is essential for developers to effectively manage access rights and ensure secure interactions within the Ethereum ecosystem.
Mastering the Checks-Effects-Interactions Pattern
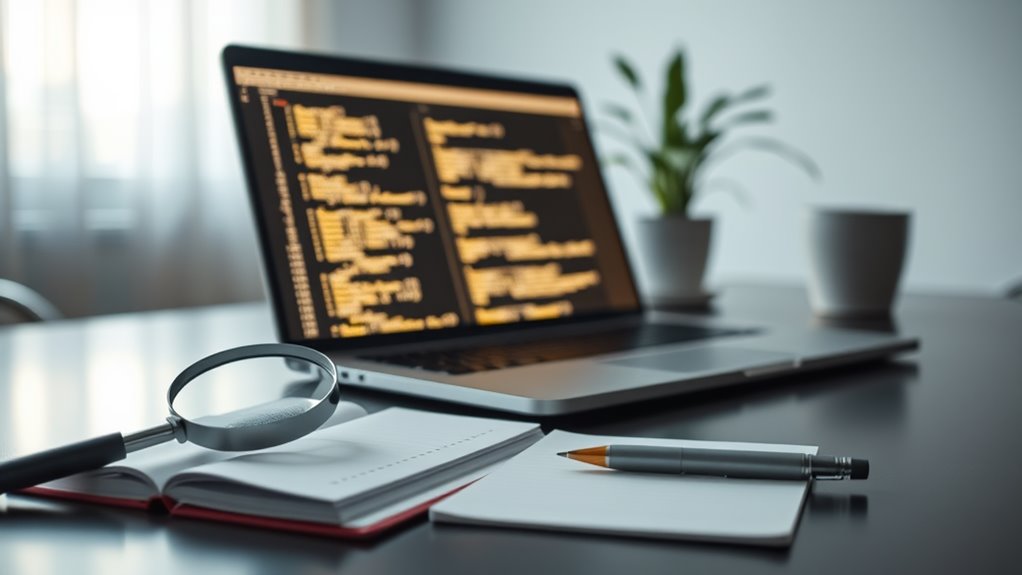
The Checks-Effects-Interactions pattern provides critical protection for contracts handling financial transactions.
When implementing fund transfers, developers should structure code to validate conditions first, update internal states second, and perform external calls last.
This ordering effectively prevents reentrancy attacks, as demonstrated by numerous case studies where proper implementation has safeguarded millions in cryptocurrency assets. Additionally, ensuring transparency about the project team can help prevent potential scams and protect investors from losses.
Implementation Cases
When developers implement the Checks-Effects-Interactions pattern correctly, they create robust smart contracts that resist common vulnerabilities like reentrancy attacks.
This pattern guarantees that transactions follow a secure sequence of operations, protecting users’ assets and maintaining contract integrity.
Common implementation cases include:
- Token withdrawal functions that update balances before transferring funds
- Marketplace contracts that change ownership records before sending payments
- Auction systems that finalize winning bids in state before notifying participants
- Lending platforms that adjust collateral ratios before initiating liquidations
- Governance contracts that record votes before executing approved proposals
Securing Fund Transfers
Mastering the Checks-Effects-Interactions pattern represents a critical skill for developers seeking to protect their smart contracts from malicious attacks. This security design principle guarantees that state variables are updated before any external calls occur, greatly reducing reentrancy vulnerabilities that could lead to asset theft.
The 2016 DAO hack dramatically demonstrated the consequences of ignoring this pattern, resulting in millions of dollars lost. When implementing fund transfers, developers should follow the CEI sequence: first verify conditions, then modify contract state, and only afterward interact with external contracts. This ordering prevents attackers from exploiting incomplete state changes during execution.
For additional protection, developers can implement mutex locks to further secure high-value transactions and conduct thorough testing in environments like Sepolia before mainnet deployment.
Selecting Trusted External Code Libraries and Dependencies
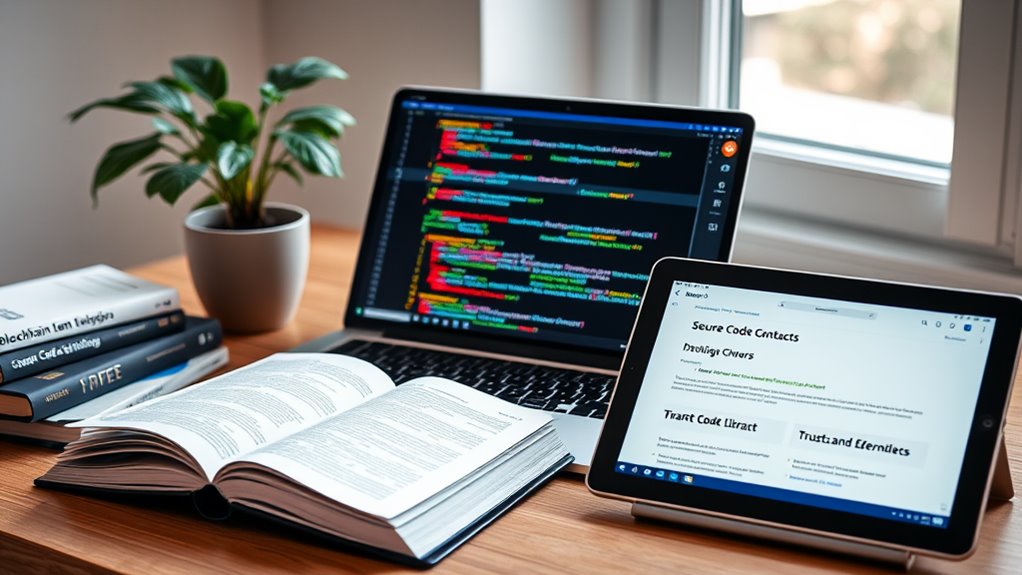
Successful implementation of secure smart contracts depends heavily on the quality and reliability of external code libraries and dependencies integrated into the project. Developers must carefully evaluate these components to prevent vulnerabilities from compromising their applications.
- Use libraries from reputable sources with strong community validation and regular maintenance.
- Verify that external dependencies have undergone thorough security audits by recognized experts.
- Prefer immutable contracts with publicly available source code for transparency.
- Implement standardized interfaces like ERC-20 to guarantee compatibility and reduce security risks.
- Establish a monitoring system to track security updates and potential vulnerabilities in dependencies.
When integrating external code, following the Checks-Effects-Interactions pattern and implementing extensive error handling mechanisms will considerably reduce exploitation risks while maintaining peak performance. Moreover, the integration of artificial intelligence is becoming essential for detecting threats in real-time and enhancing security measures.
Conducting Comprehensive Security Audits Before Deployment
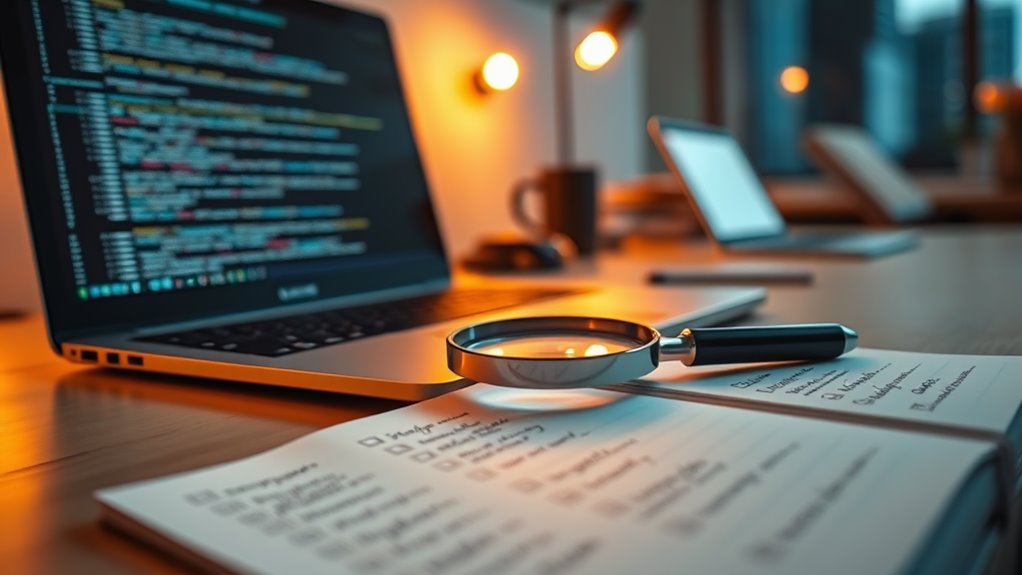
Security audits represent the critical final checkpoint before smart contracts are permanently deployed to the blockchain, where their immutable nature makes post-deployment fixes costly or impossible.
These audits combine automated and manual reviews to identify vulnerabilities that could compromise assets or functionality.
A thorough audit process begins with documentation gathering, followed by automated analysis using specialized tools like Slither and Mythril.
Expert reviewers then conduct manual code examinations to catch issues that automated tools might miss.
The audit team collaborates with developers to address identified vulnerabilities before finalizing a detailed report.
Beyond code review, extensive audits should evaluate surrounding infrastructure, key management procedures, and potential attack vectors like reentrancy.
This multi-layered approach builds stakeholder trust while potentially saving projects from devastating security breaches. Additionally, implementing two-factor authentication can significantly enhance security measures against unauthorized access during the audit process.
Building Smart Contracts With Robust Upgrade Paths
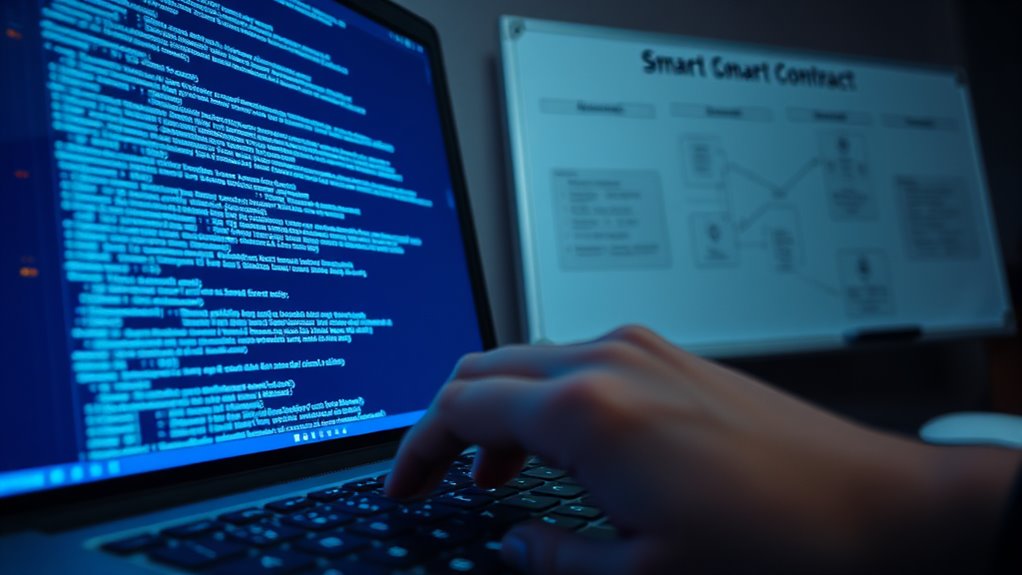
Smart contracts should be designed with mutability in mind, allowing for updates that address vulnerabilities or add functionality while maintaining data integrity.
Implementing a robust governance mechanism guarantees that upgrades are conducted transparently and with proper stakeholder input, reducing the risk of centralized control.
These upgrade paths, when properly constructed, create resilient smart contracts that can evolve with changing security requirements and technical advancements.
Design for Mutability
Building smart contracts with mutability in mind represents a critical approach to blockchain development, as it guarantees systems can adapt to changing requirements and security challenges over time.
Developers implementing upgradeable contracts markedly reduce the risks associated with immutability while preserving blockchain’s core benefits.
- Proxy patterns allow seamless updates without changing contract addresses, maintaining continuity for users.
- Diamond proxies provide solutions for complex contracts that exceed size limits while enhancing flexibility.
- Gradual release strategies enable testing changes in phases before full implementation.
- Multiple upgrade mechanisms create versatility for handling different scenarios and requirements.
- Planning for upgrades from the outset guarantees smoother shifts when modifications become necessary.
This approach balances blockchain’s permanence with practical needs for adaptation, helping projects evolve while protecting user assets and maintaining trust.
Governance Mechanism Implementation
While blockchain technologies continue to evolve, implementing robust governance mechanisms has become essential for managing smart contract lifecycles and guaranteeing proper upgrade paths. Effective governance allows projects to adapt while maintaining security and transparency.
Well-designed governance typically features voting systems using governance tokens, with clear approval thresholds to guarantee community support. Many projects implement multisignature wallets that distribute authority, reducing single points of failure.
These systems often include timelock mechanisms that provide stakeholders time to review proposed changes before implementation.
For maximum security, governance implementations should undergo thorough auditing and include defined roles and responsibilities. The best systems balance on-chain automation with documented off-chain processes, creating a framework that can scale with project growth while protecting against unauthorized modifications or security exploits. Additionally, decentralized systems enhance security by distributing data and control across multiple nodes, thereby fortifying governance mechanisms against potential threats.
Mitigating Oracle Risks Through Multiple Data Sources
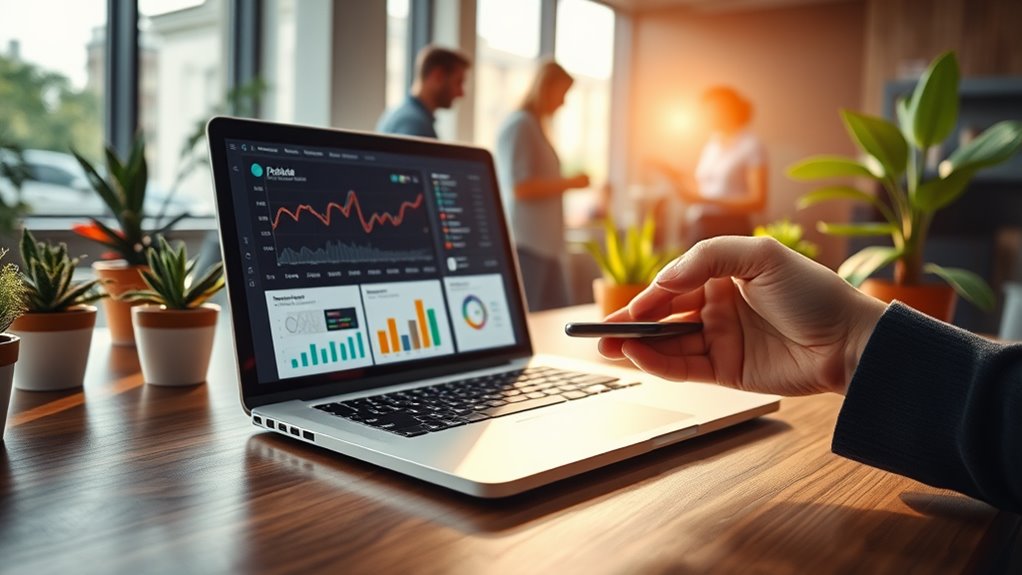
Decentralized oracle networks represent one of the most effective strategies for mitigating smart contract risks related to external data. By utilizing multiple data sources and verification nodes, smart contracts can avoid manipulation risks and maintain data accuracy even if individual sources fail or become compromised.
- Implement median calculations to eliminate outliers and prevent manipulation attempts
- Diversify oracle providers to reduce dependency on any single service or infrastructure
- Establish cross-verification mechanisms between independent data sources
- Prioritize oracle solutions with robust encryption and secure data transmission
- Conduct regular audits of oracle integration to identify and address potential vulnerabilities
These measures greatly reduce the likelihood of oracle failures affecting smart contract execution, enhancing overall system reliability while preserving the trustless nature of blockchain applications.
Leveraging Automated Testing Tools for Vulnerability Detection
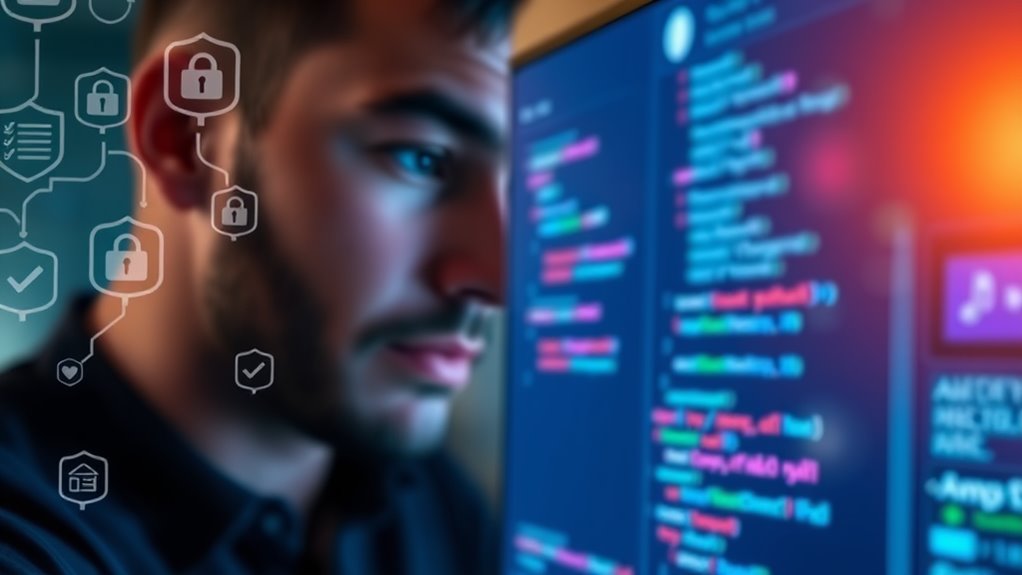
Automated security tools form the backbone of modern smart contract security practices, offering developers a systematic approach to identify vulnerabilities before deployment. These tools employ various techniques to detect potential weaknesses, each serving specific security needs.
Static analysis tools like Slither and SmartCheck examine code without execution, while symbolic execution tools such as MythX and Manticore explore possible execution paths to identify vulnerabilities like reentrancy attacks.
For runtime testing, fuzzing tools like Echidna generate random inputs to uncover edge cases.
Experts recommend implementing multiple tools in continuous integration pipelines, combining static and dynamic approaches for thorough coverage.
Despite their effectiveness, automated tools should complement rather than replace manual audits, as complex logic errors often require human expertise to identify and address.
Integrating AI and Machine Learning for Enhanced Smart Contract Security
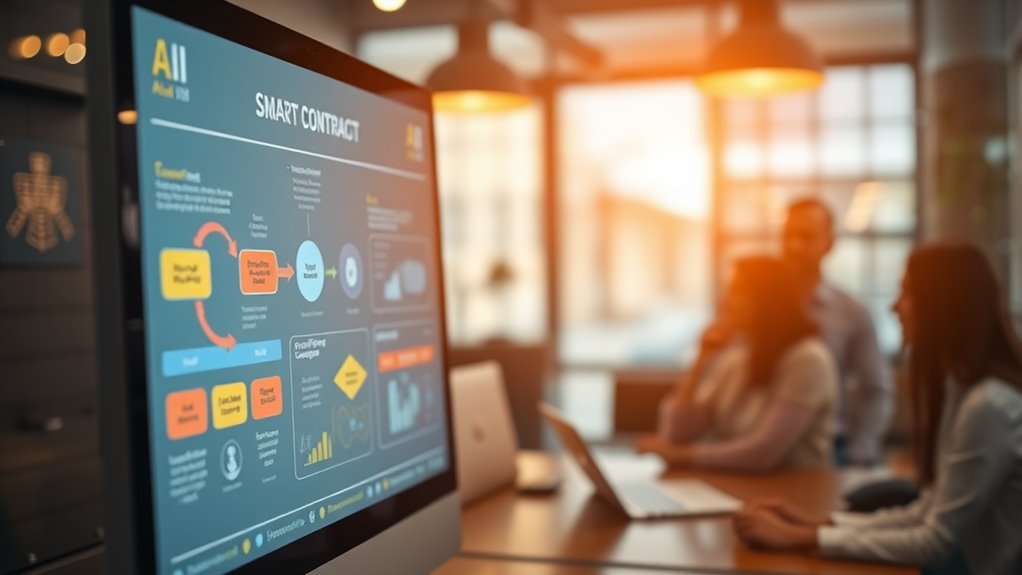
Artificial intelligence and machine learning have revolutionized smart contract security, offering capabilities that extend beyond traditional automated testing tools. These technologies analyze complex patterns in code and transactions, identifying vulnerabilities that might otherwise go undetected.
- Graph neural networks examine temporal message propagation to uncover hidden security flaws.
- Real-time transaction monitoring flags suspicious activities instantly, improving response times.
- Predictive analytics use historical data to forecast potential security breaches before they occur.
- Multi-agent reinforcement learning simulates various attack scenarios to test contract robustness.
- Convolutional layer analysis captures detailed patterns in source code for thorough vulnerability detection.
As these AI technologies continue to evolve, they provide increasingly sophisticated protection against emerging threats while reducing the need for manual oversight and improving regulatory compliance.
Frequently Asked Questions
Can Smart Contract Insurance Protect Against Financial Losses From Vulnerabilities?
Smart contract insurance can provide financial protection against losses from vulnerabilities, but it doesn’t prevent the vulnerabilities themselves. It offers compensation when exploits occur, functioning as a safety net for affected parties.
How Do Gas Fees Affect Smart Contract Security Design Choices?
Gas fees incentivize efficient coding practices, as developers must balance security features against transaction costs. Higher complexity increases expenses, potentially leading to compromises in security implementation to maintain economic viability for users.
What Privacy Concerns Arise From Implementing Multi-Signature Mechanisms?
Like a transparent window into private affairs, multi-signature mechanisms expose transaction structures on the blockchain, risk revealing group identities, challenge regulatory compliance, and create complex key management issues requiring robust privacy-preserving techniques.
Can Quantum Computing Crack Current Smart Contract Security Measures?
Yes, quantum computers could eventually crack current smart contract security measures. They threaten cryptographic algorithms like ECDSA and RSA through Shor’s Algorithm, necessitating the adoption of quantum-resistant cryptography to maintain blockchain security.
How Can Developers Balance Security Features With User Experience?
Like architects blending fortresses with comfort, developers balance security and user experience by implementing modular designs, role-based access controls, optimizing gas usage, and conducting regular audits while maintaining intuitive interfaces and clear documentation.
Conclusion
Smart contract security remains an ongoing journey rather than a destination. By implementing these protective measures, developers can considerably reduce the likelihood of unwanted surprises. While no code can be guaranteed completely bulletproof, these practices create robust digital agreements that stand up to scrutiny. The evolving landscape of blockchain security demands vigilance, but with proper precautions, smart contracts can fulfill their revolutionary potential.